<WSL>VSCode, Debug 환경설정
Pre-Work.
더보기
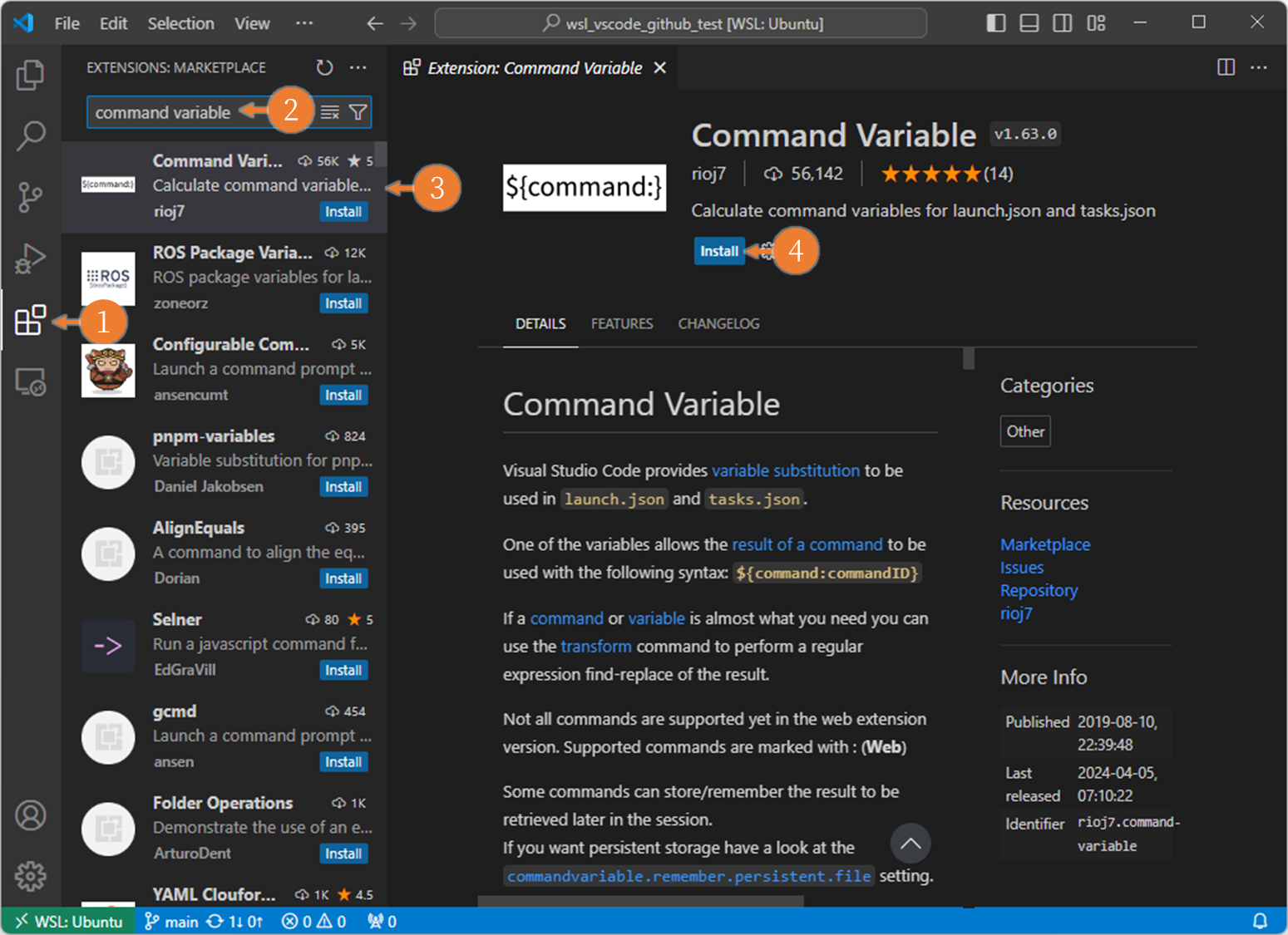
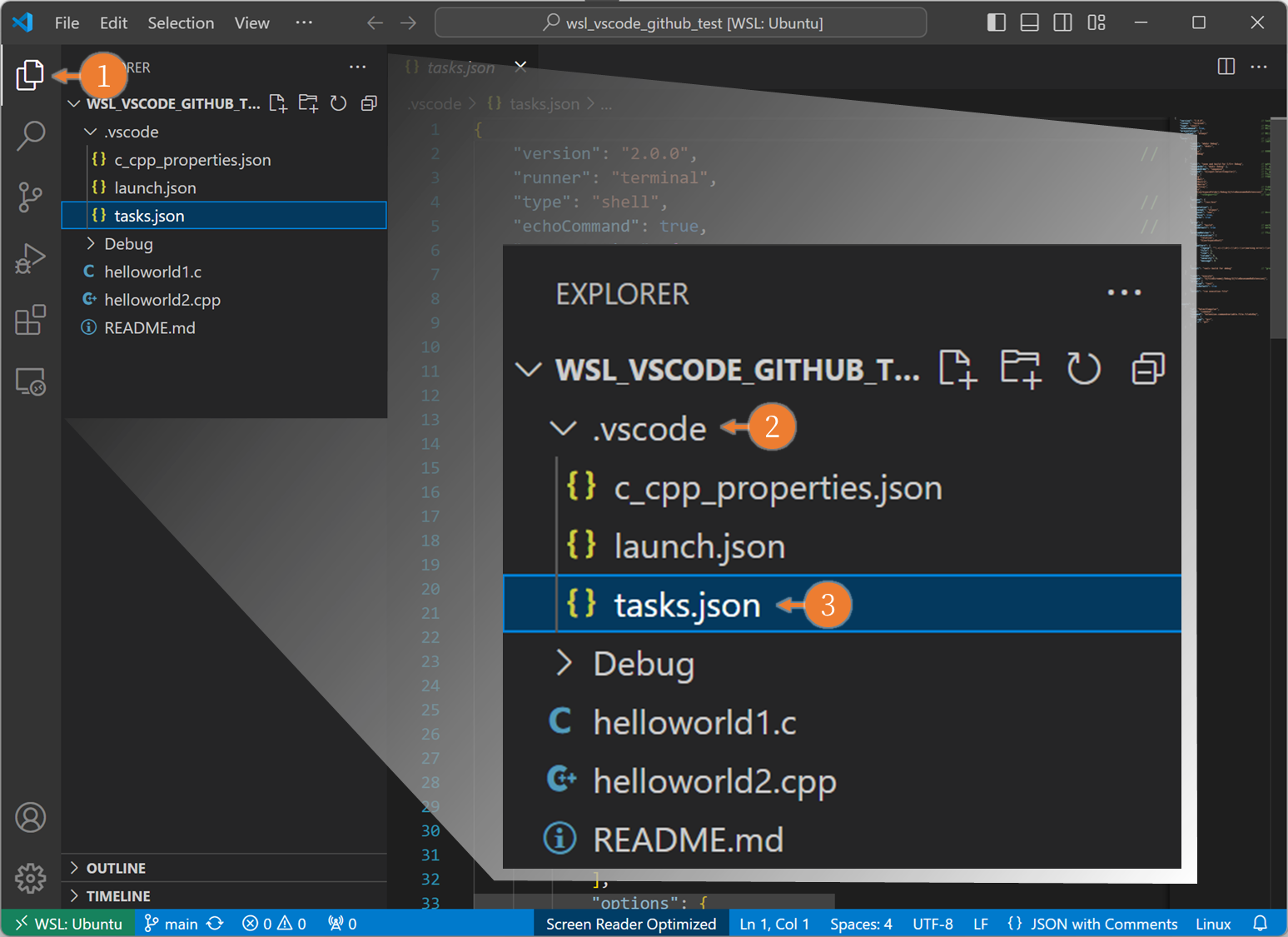
GDB(GNU DeBugger) 설치는 필수입니다.
01. WSL 환경에서 VS Code, C/C++ 환경설정
<WSL> VS Code, C/C++ 환경설정
MSDN 공식문서, VSCode 공식문서 WSL 환경설정더보기01. WSL 설치 WSL2 환경 설정[WSL 공식문서], [WSL 개발 환경 설정]Step1. 윈도우에서 WSL 활성화더보기Window + S 단축키를 누르면, 검색 기능이 활성화 됩
basiclike.tistory.com
02. VS code Extensions 에서 Command Variable 설치
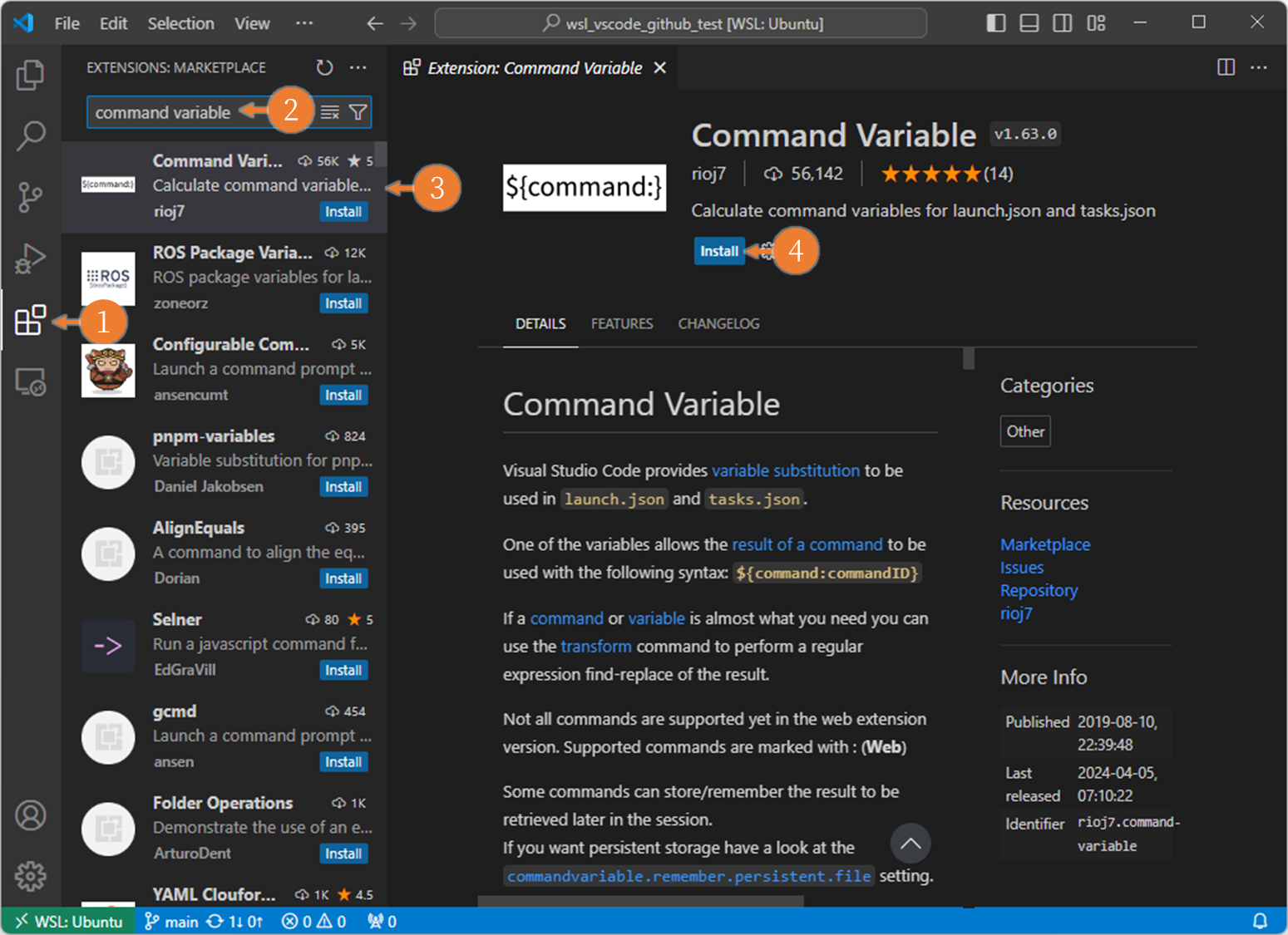
03. task.json 파일을 수정합니다.
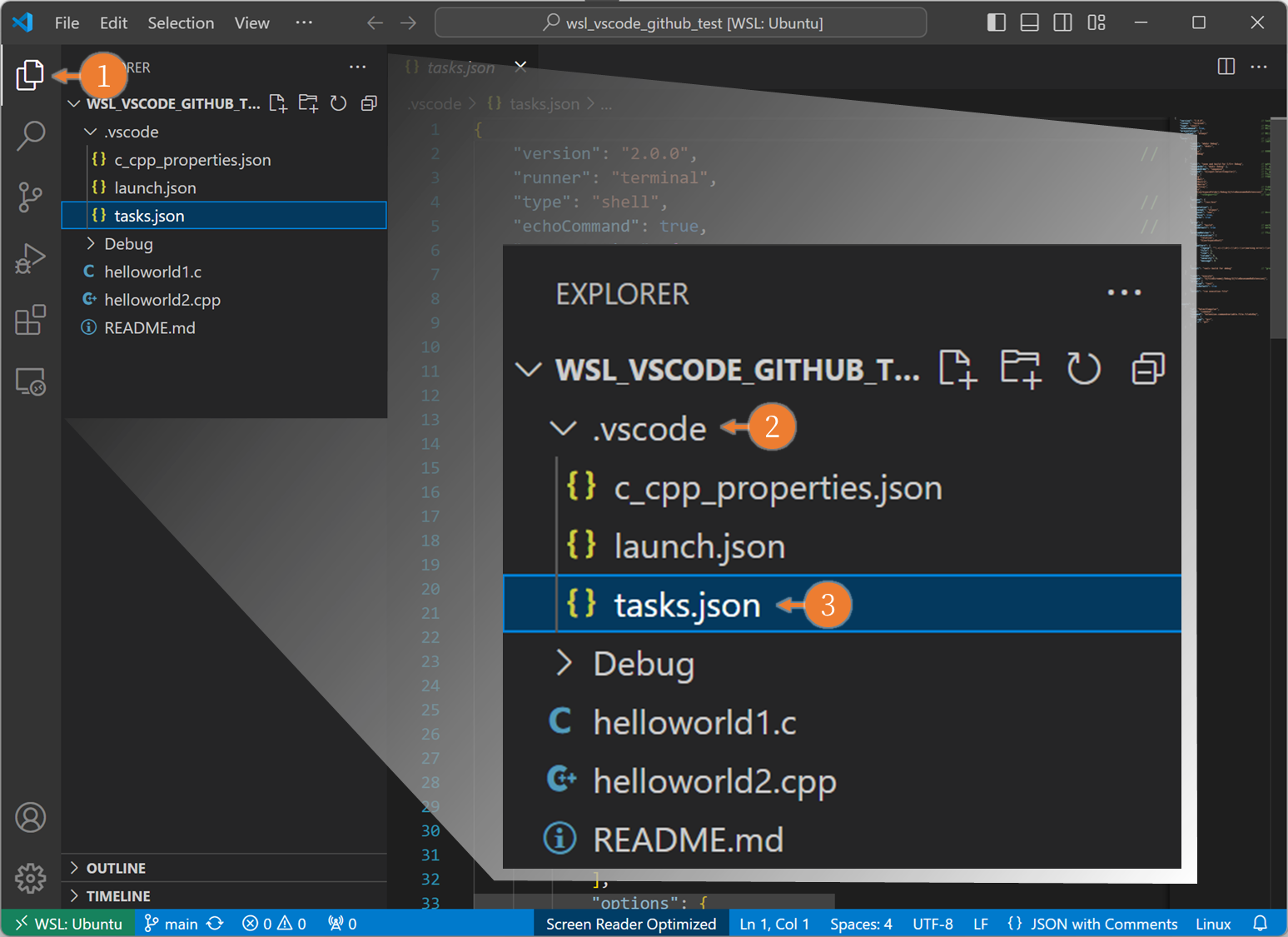
{
"version": "2.0.0", // task.json 파일 형식 버전
"runner": "terminal",
"type": "shell", // 터미널 유형: process, shell, cppbuild
"echoCommand": true, // 실행 명령 터미널 출력 여부
"presentation": {
"reveal": "always" // 실행 중 출력 창 항상 표시 여부
},
"tasks": [ // 빌드 작업 실행할 목록 작성
{ // [작업 이름] make Debug directory
"label": "mkdir Debug",
"command": "mkdir",
"args": [
"-p", // 하위 경로에 Debug 와 동일 디렉토리 없을때만 생성하는 mkdir 명령 옵션
"Debug"
]
},
{
"label": "save and build for C/C++ Debug", // 작업명
"dependsOn":[ "mkdir Debug" ], // 1. 작업공간 하위 경로에 Debug 폴더 만들기
"dependsOrder": "sequence", // 명령 순차 실행
"command": "${input:SelectCompiler}", // 2. gcc/g++ 컴파일러 선택
"args": [ // 컴파일러에 넘길 매개변수
"-g", // 디버그 정보 포함 옵션
"-Wall",
"-Wextra",
"-Werror",
"${file}", // 소스파일
"-o", // Output 출력파일 = 실행파일
"${workspaceFolder}/Debug/${fileBasenameNoExtension}" // 빌드 결과 파일의 저장 경로와 파일명 형식
//, "-std=gnu++11" // (opt) 특정 컴파일러 버전 선택시 지정
],
"options": {
"cwd": "/usr/bin"
},
"presentation": {
"reveal": "always",
"panel": "new", // 새로운 출력 패널에 결과 표시
"focus": true,
"echo": true
},
"group": {
"kind": "build", // workbench.action.tasks.build 그룹에서
"isDefault": true // default build group task 설정, 자동 실행
},
"problemMatcher": { // 컴파일러마다 다른 Warning, Error 출력 규칙
"fileLocation": [
"relative",
"${workspaceRoot}"
],
"pattern": {
"regexp": "^(.*):(\\d+):(\\d+):\\s+(warning error):\\s+(.*)$",
"file": 1,
"line": 2,
"column": 3,
"severity": 4,
"message": 5
}
},
"detail": "<wsl> build for debug" // "group": "isDefault": true 속성이 아니면, 팔렛에서 작업의 설명으로 출력
},
{
"label": "execute",
"command": "${fileDirname}/Debug/${fileBasenameNoExtension}",
"group": {
"kind": "test",
"isDefault": true
},
"detail": "run execution file"
}
],
"inputs": [
{
"id": "SelectCompiler",
"type": "command",
"command": "extension.commandvariable.file.fileAsKey",
"args": {
".cpp": "g++",
".c": "gcc"
}
}
]
}
Step 1. launch.json 설정
더보기
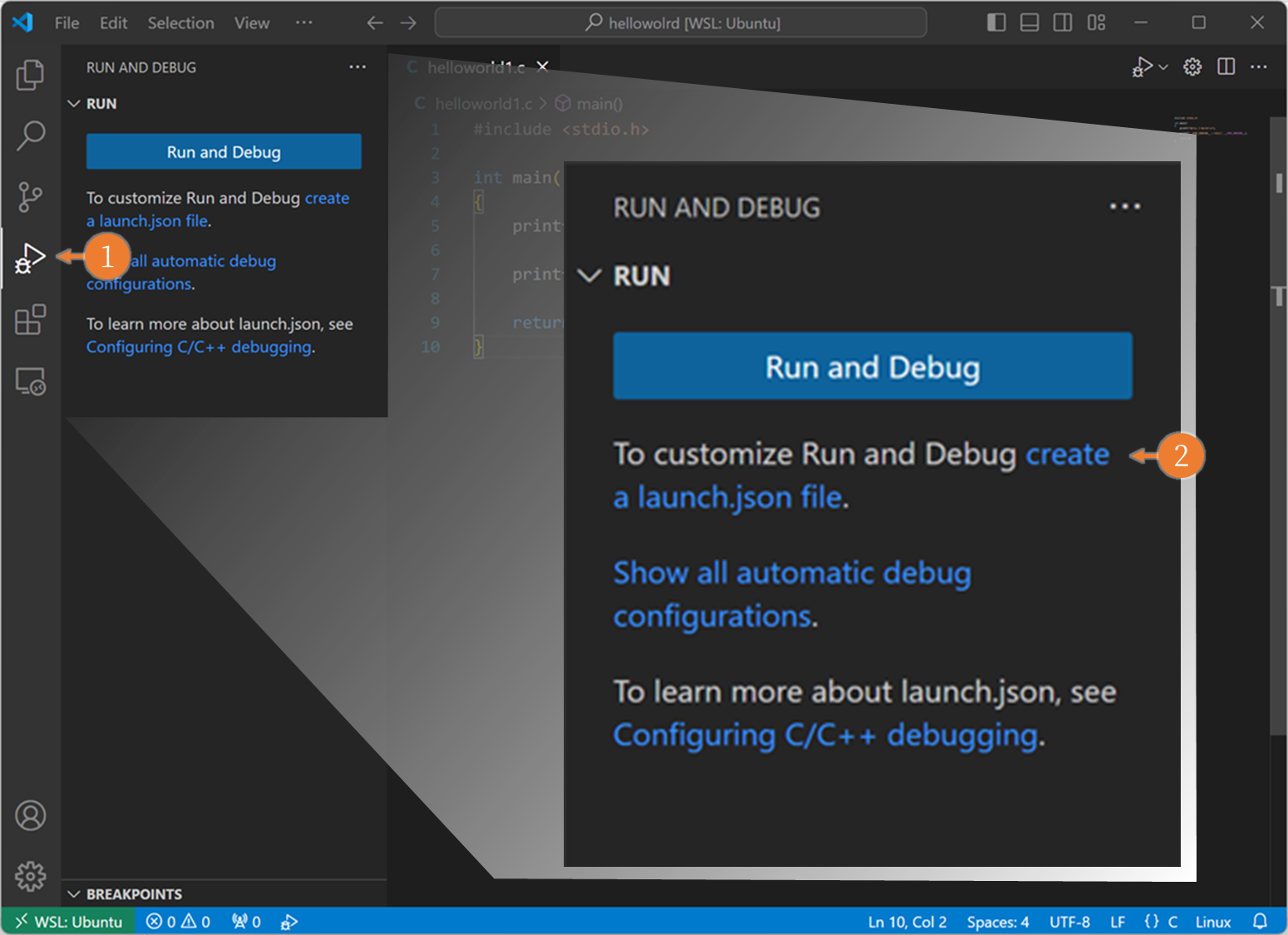
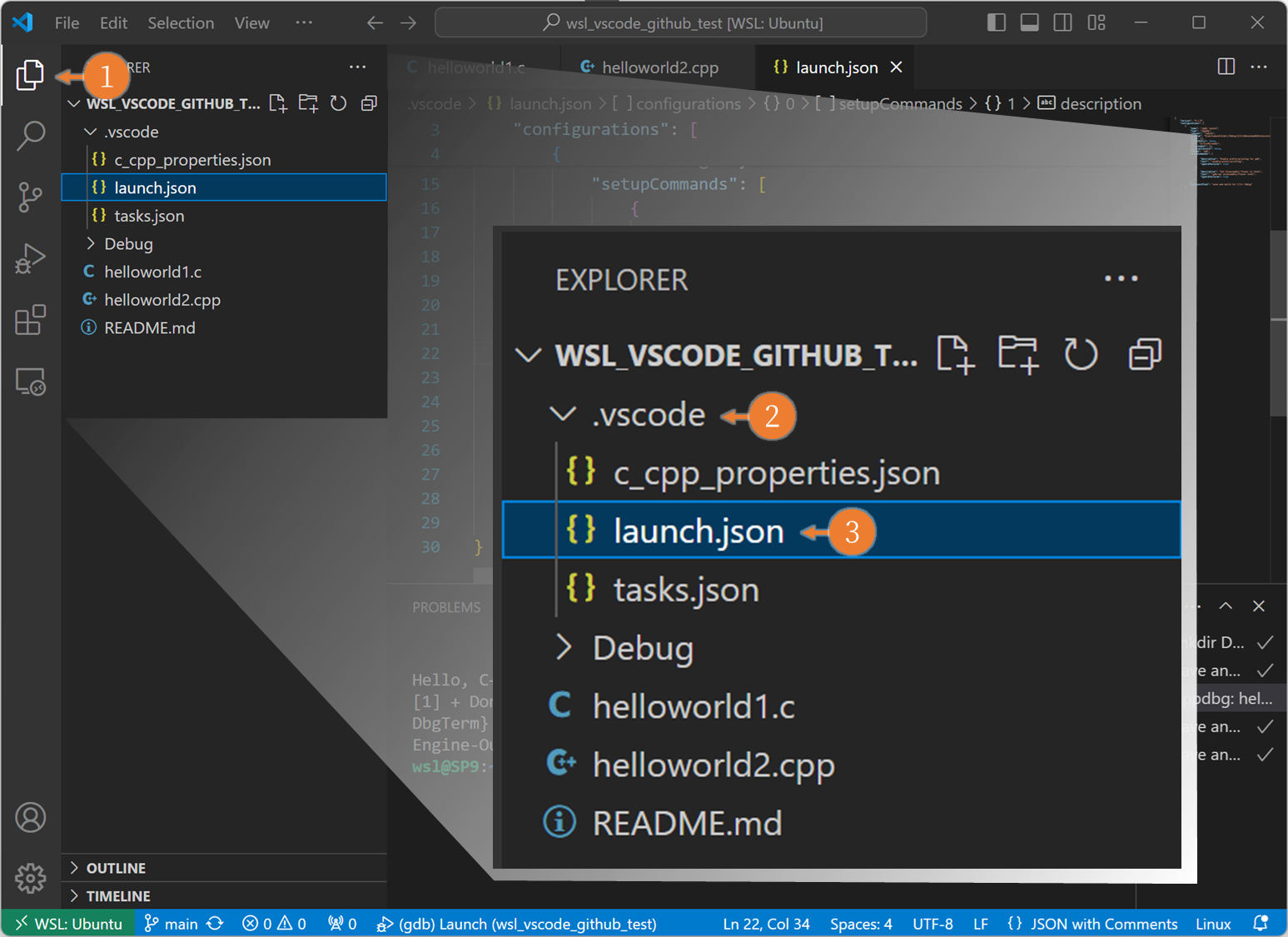
01. launch.json 만들기
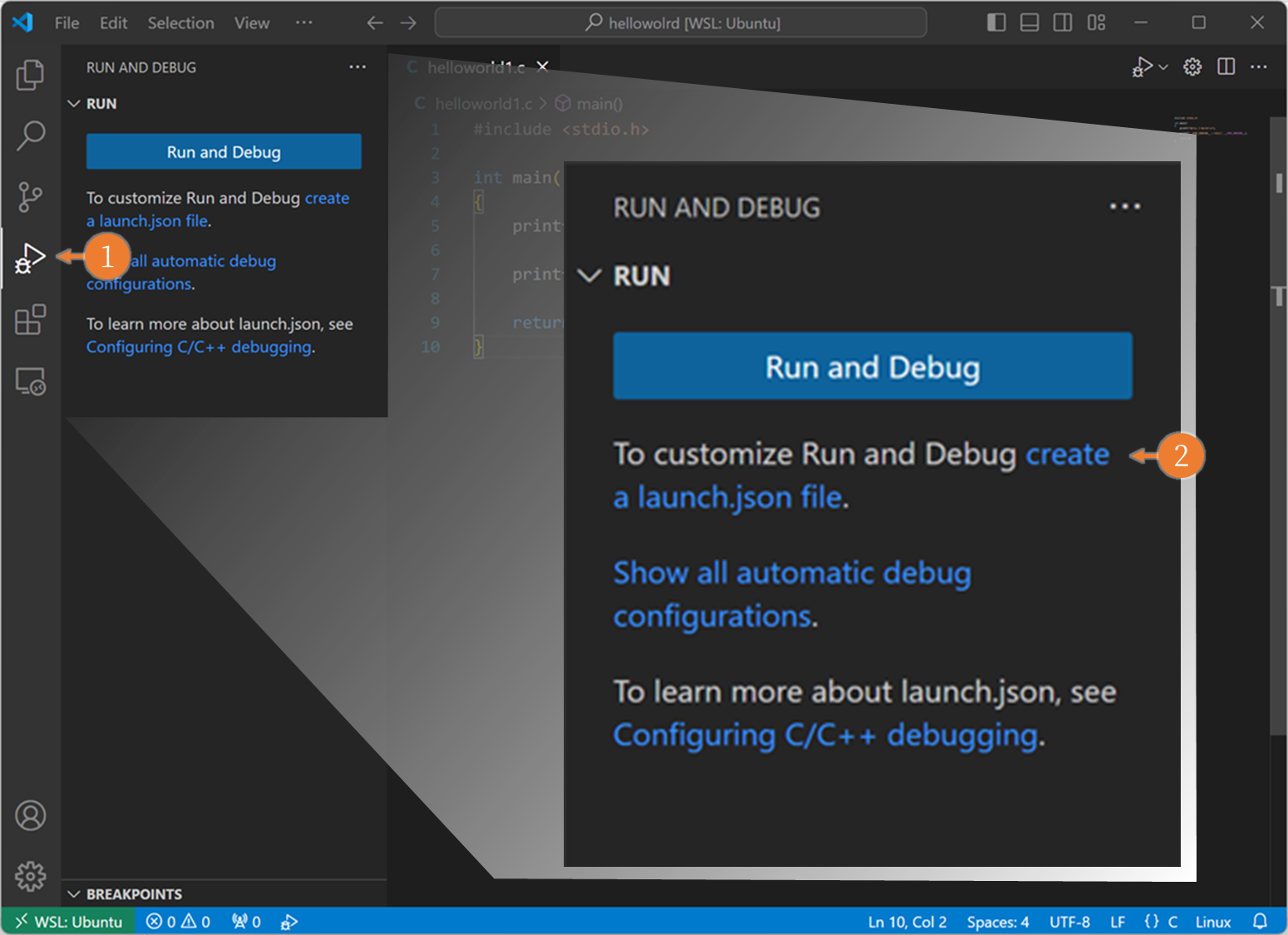
02. launch.json 수정
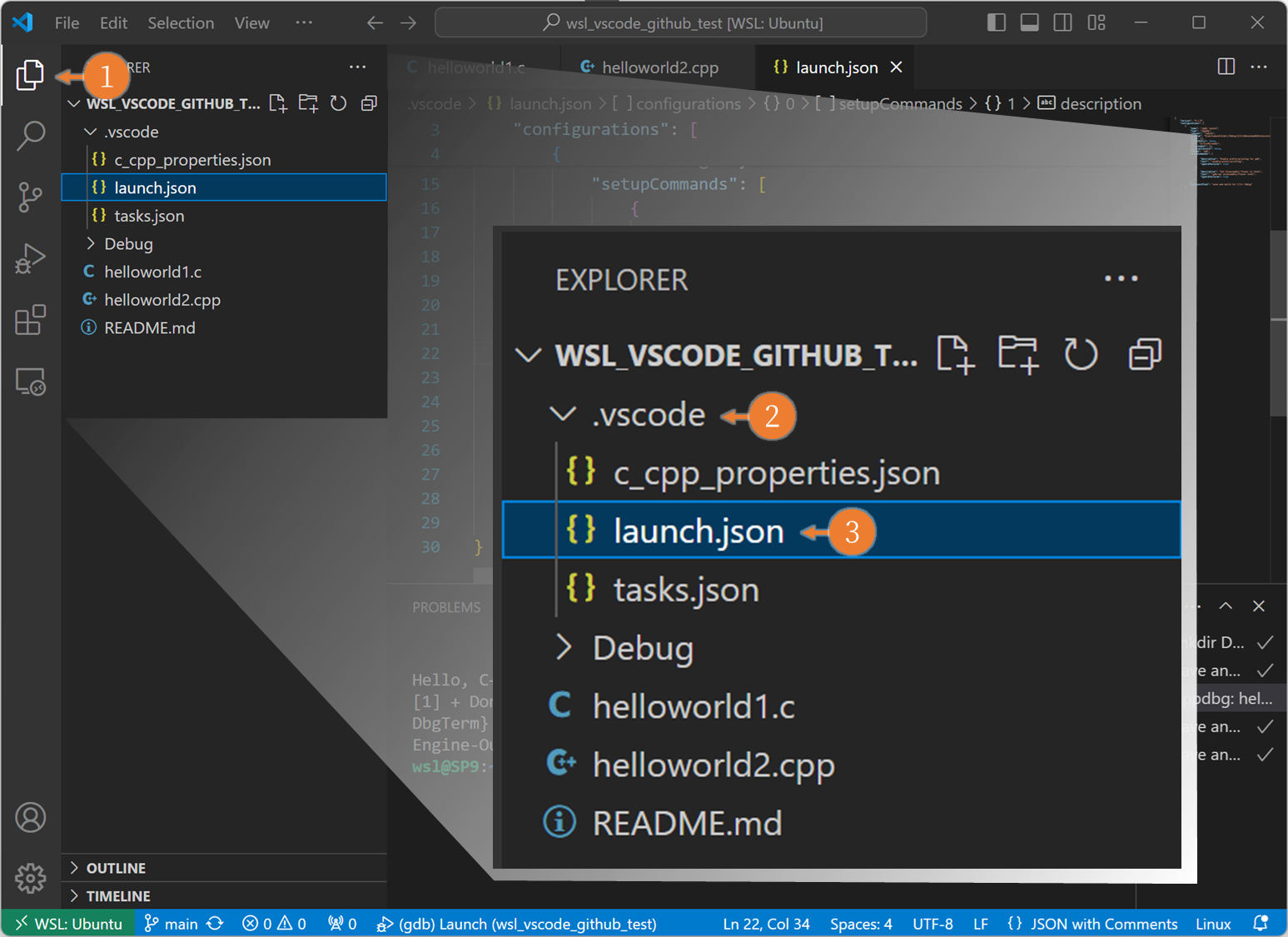
{
"version": "0.2.0",
"configurations": [
{
"name": "(gdb) Launch", // debug 작업 이름
"type": "cppdbg", // debug type
"request": "launch", // debug request
"program": "${workspaceFolder}/Debug/${fileBasenameNoExtension}", // launch 하는 대상, 실행 프로그램
"args": [], // 실행 프로그램에 대해 전달할 인자가 필요한 경우
"stopAtEntry": false, // debug 시작 후, main 시작에서 정지 여부
"cwd": "${fileDirname}", // 작업 경로
"environment": [], // 환경 변수 설정
"externalConsole": false, // vscode terminal 외, 외부의 terminal 실행 여부
"MIMode": "gdb", // debugger 종류
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
},
{
"description": "Set Disassembly Flavor to Intel",
"text": "-gdb-set disassembly-flavor intel",
"ignoreFailures": true
}
],
"preLaunchTask": "save and build for C/C++ Debug" // launch 실행 전 사전작업, e.g) task.json build
}
]
}
Step 2. Debugging test
더보기
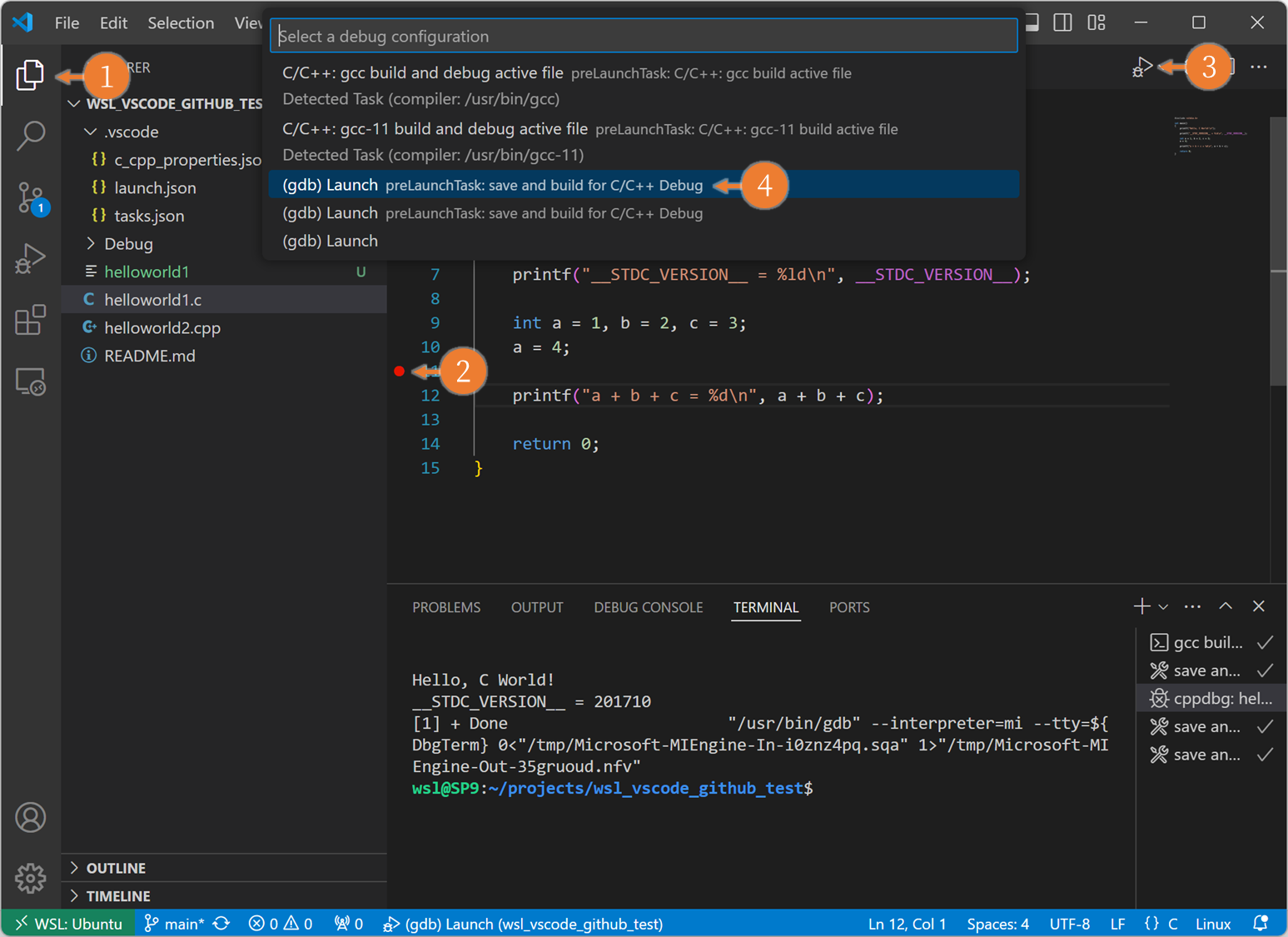
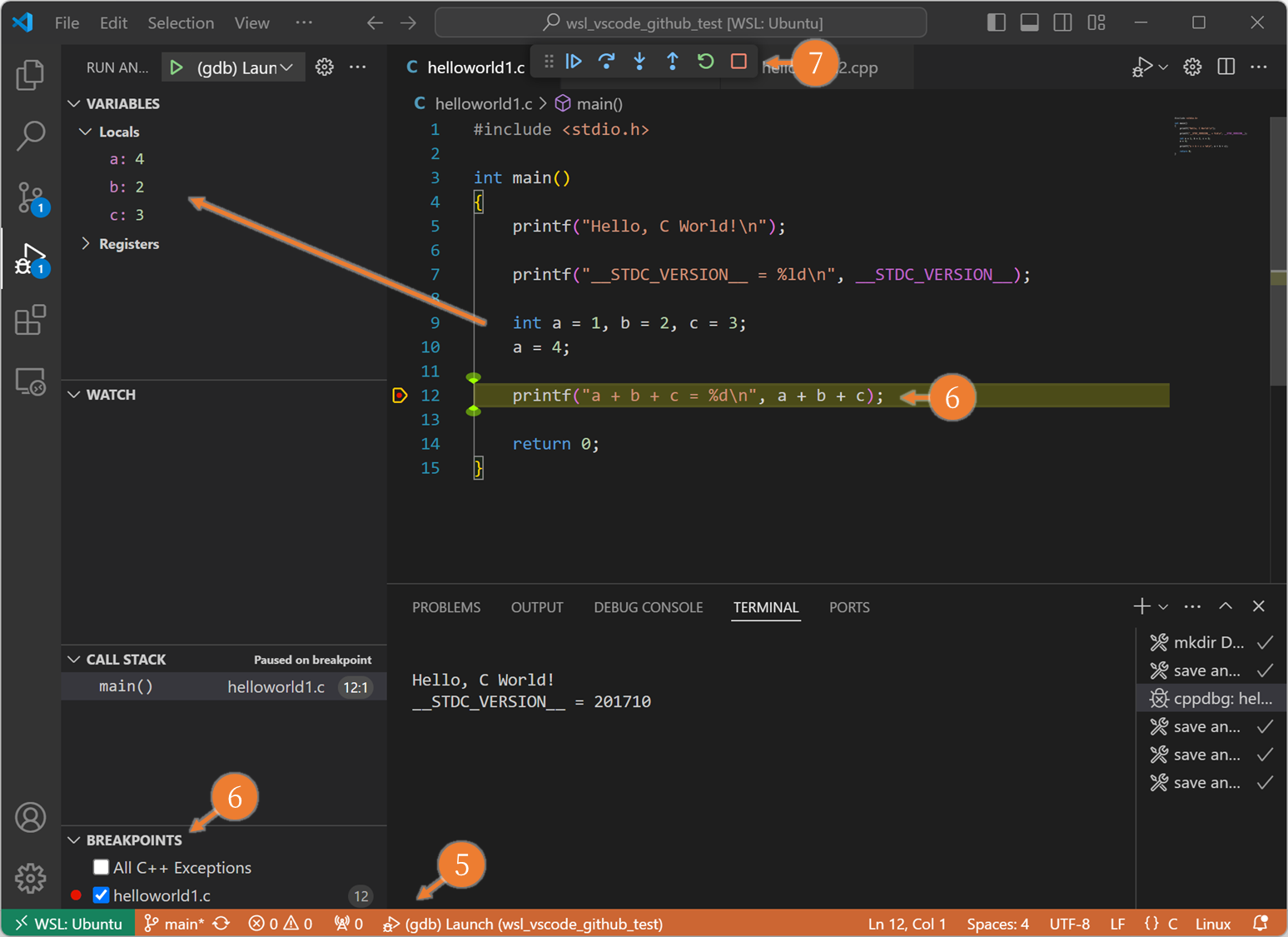
01. Test C/C++ 소스코드 준비
C test code
#include <stdio.h>
unsigned int uiSum;
int main()
{
printf("Hello, C World!\n");
printf("__STDC_VERSION__ = %ld\n", __STDC_VERSION__);
unsigned int uiCtr;
uiSum = 0;
for(uiCtr = 0; uiCtr < 100; uiCtr++)
{
uiSum += uiCtr;
}
printf("%d\n", uiSum);
return 0;
}
C++ test code
#include <stdio.h>
int main()
{
printf("Hello, C World!\n");
printf("__STDC_VERSION__ = %ld\n", __STDC_VERSION__);
int a = 1, b = 2, c = 3;
a = 4;
printf("a + b + c = %d\n", a + b + c);
return 0;
}
02. Debug
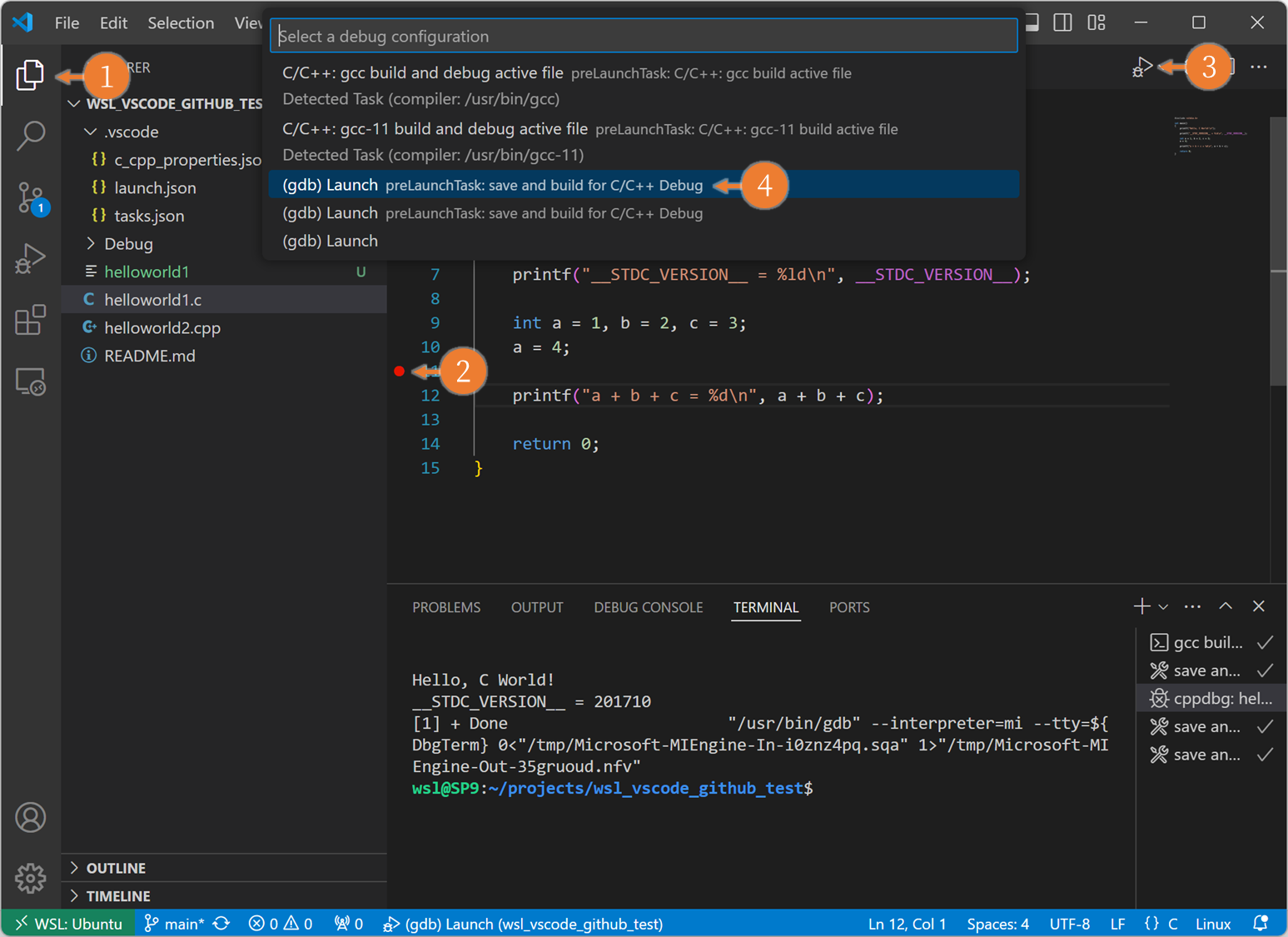
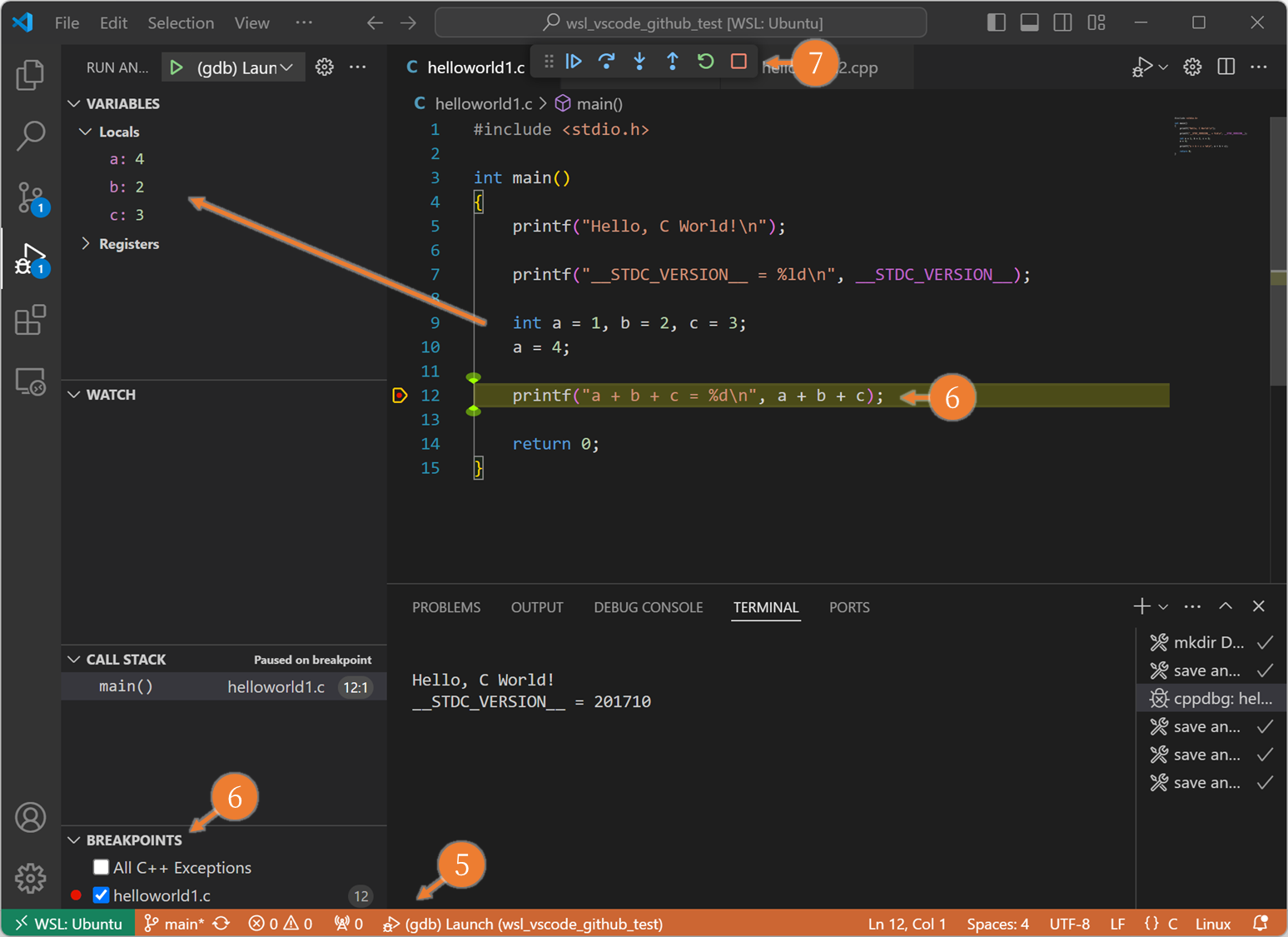
Step 3. VS Code Debug Details
더보기
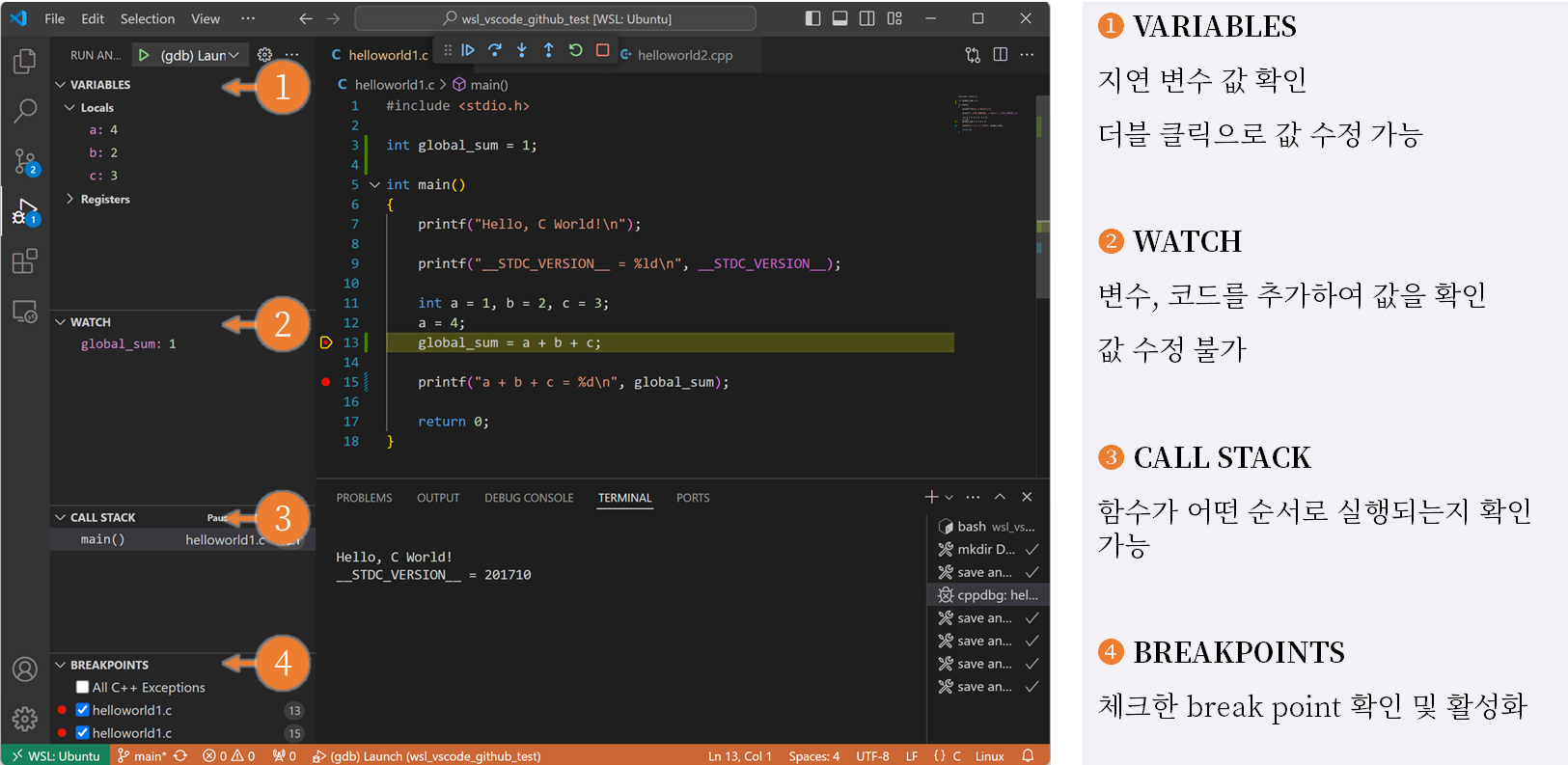

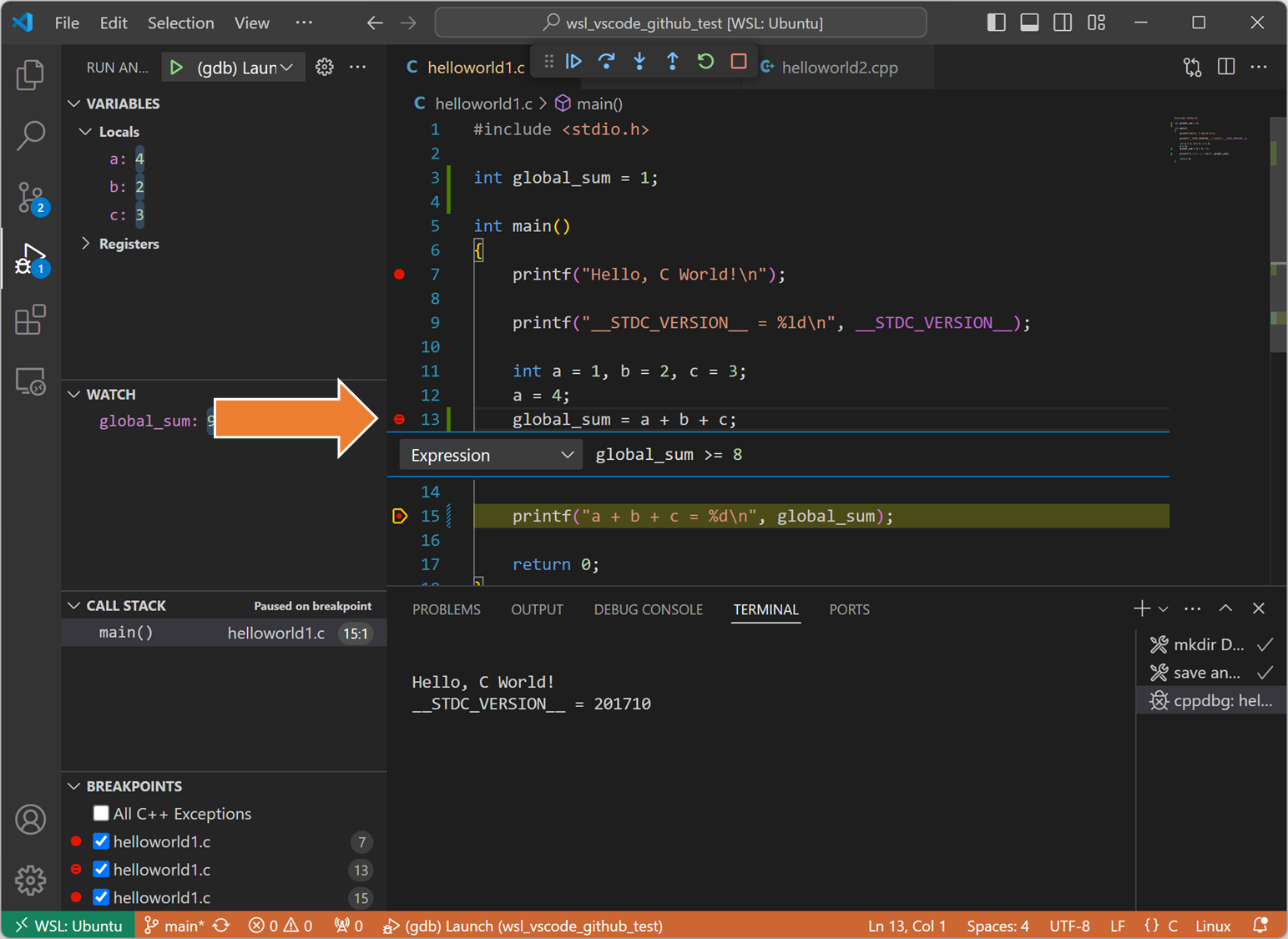
01. Debug 우측 메뉴
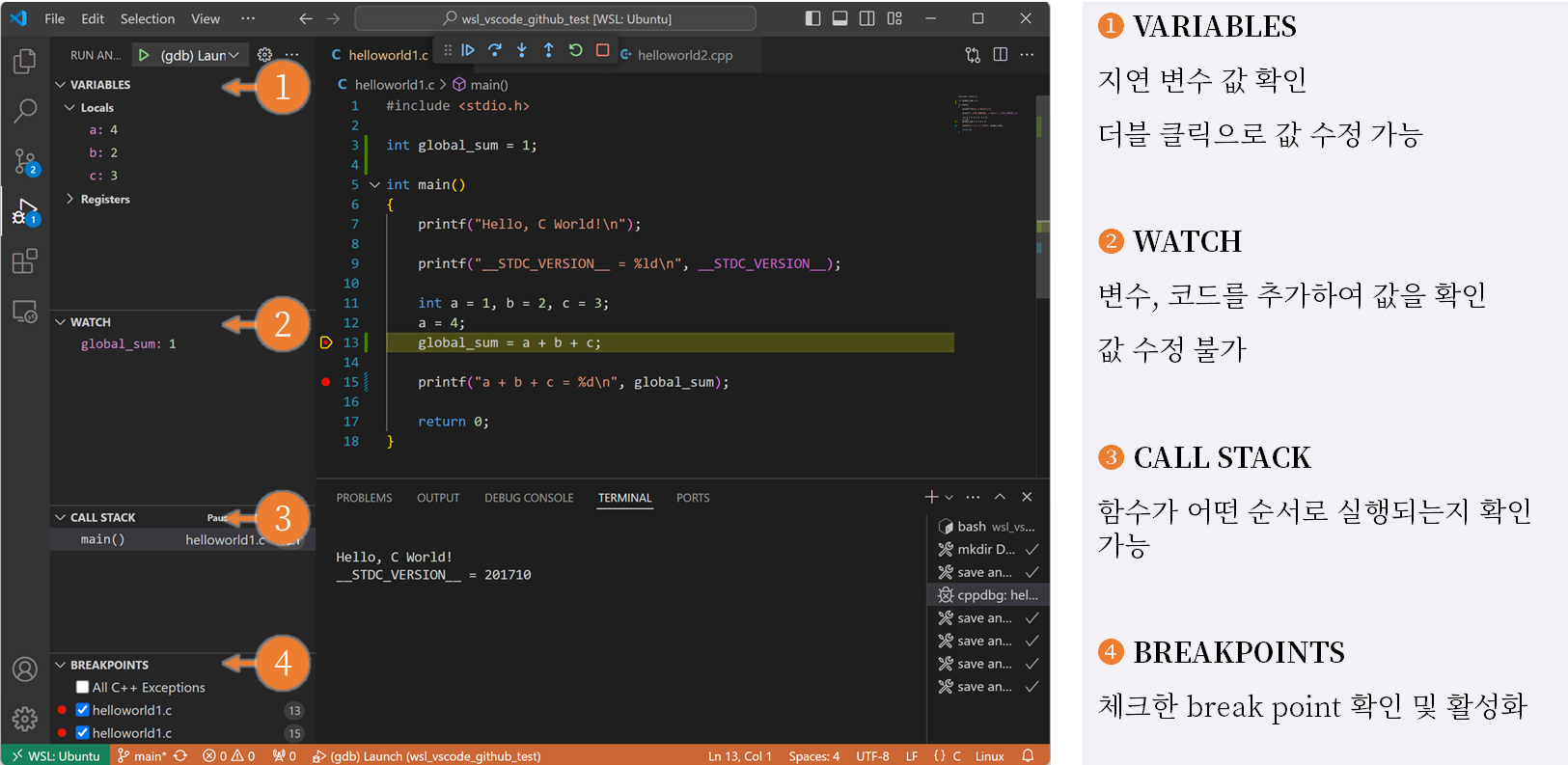
02. Debug 진행 메뉴

- Continue : 다음 break point 로 실행
- Step over : 소스코드 명령 한 줄씩 실행 (함수 안의 동작 확인하지 않음)
- Step into : 정의한 함수 안의 동작 실행
- Step out : 함수 바깥으로 이동
- Restart : 재시작
- Stop : 디버깅 중지
03. breakpoint 조건
Breakpoint 우클릭시, Edit Breakpoint 기능으로 Expression, Hit count, Log Message 중단점 조건 선택 가능
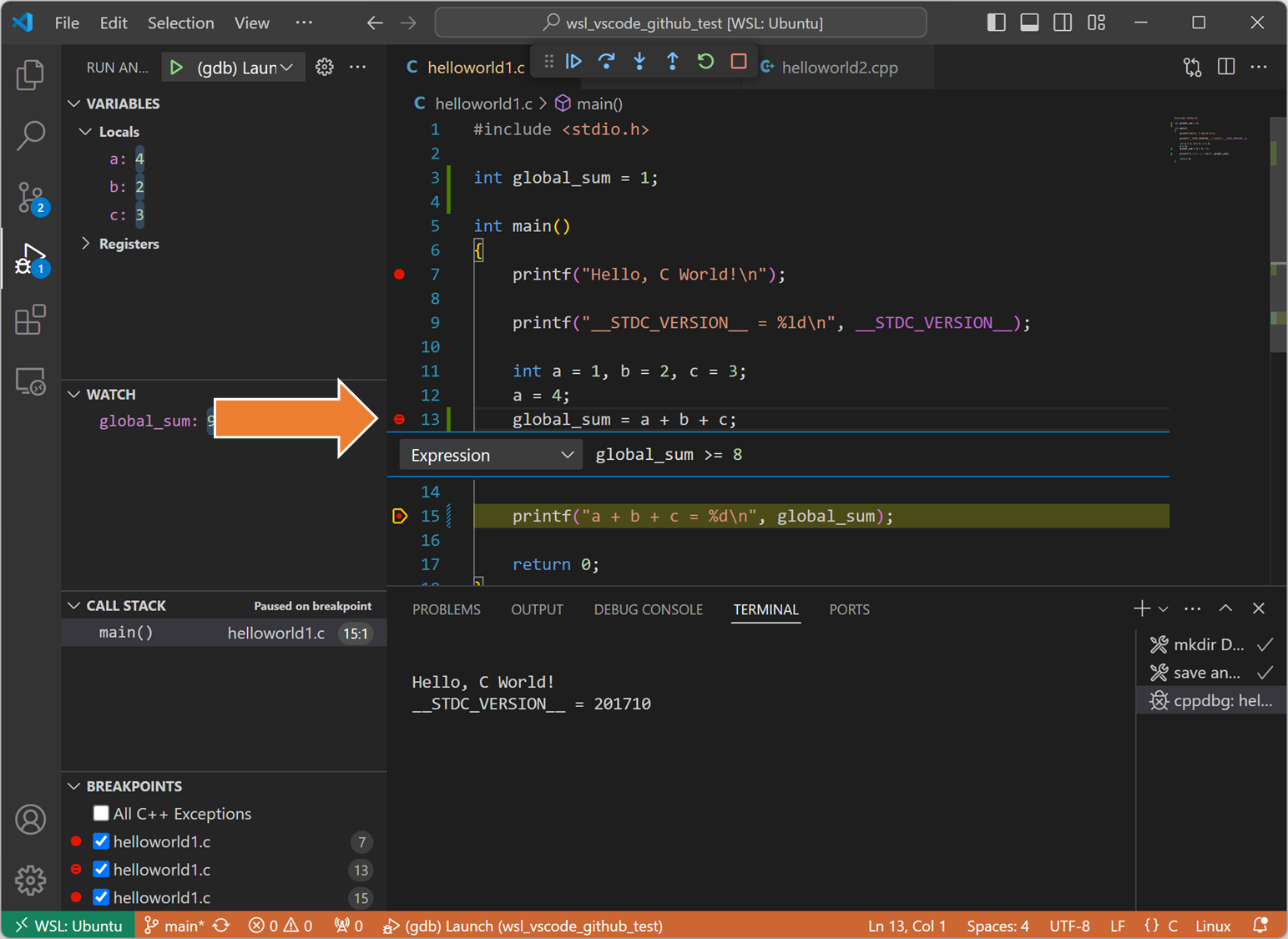