UserControl - 화면 전환
01. 목표
WPF 화면 전환에는 Page, Window, TabControl, UserControl 방법이 있습니다.
일반적인 사용 방법을 예로 들면
1) Page 는 URI로 이동할때
2) Window 는 창 위에 새 창을 띄울 때
3) TabControl 은, 탭을 사용할 때
4) UserControl 은, 화면의 일부를 구성하고 변경을 할 때 사용합니다.
이번 포스트에서는,
UserControl 을 사용해 로그인 후, 다음 화면으로 전환되도록 구현합니다.
02. LoginView 만들고 MainWindow 적용하기
02.1 MainWindow.xaml 세팅
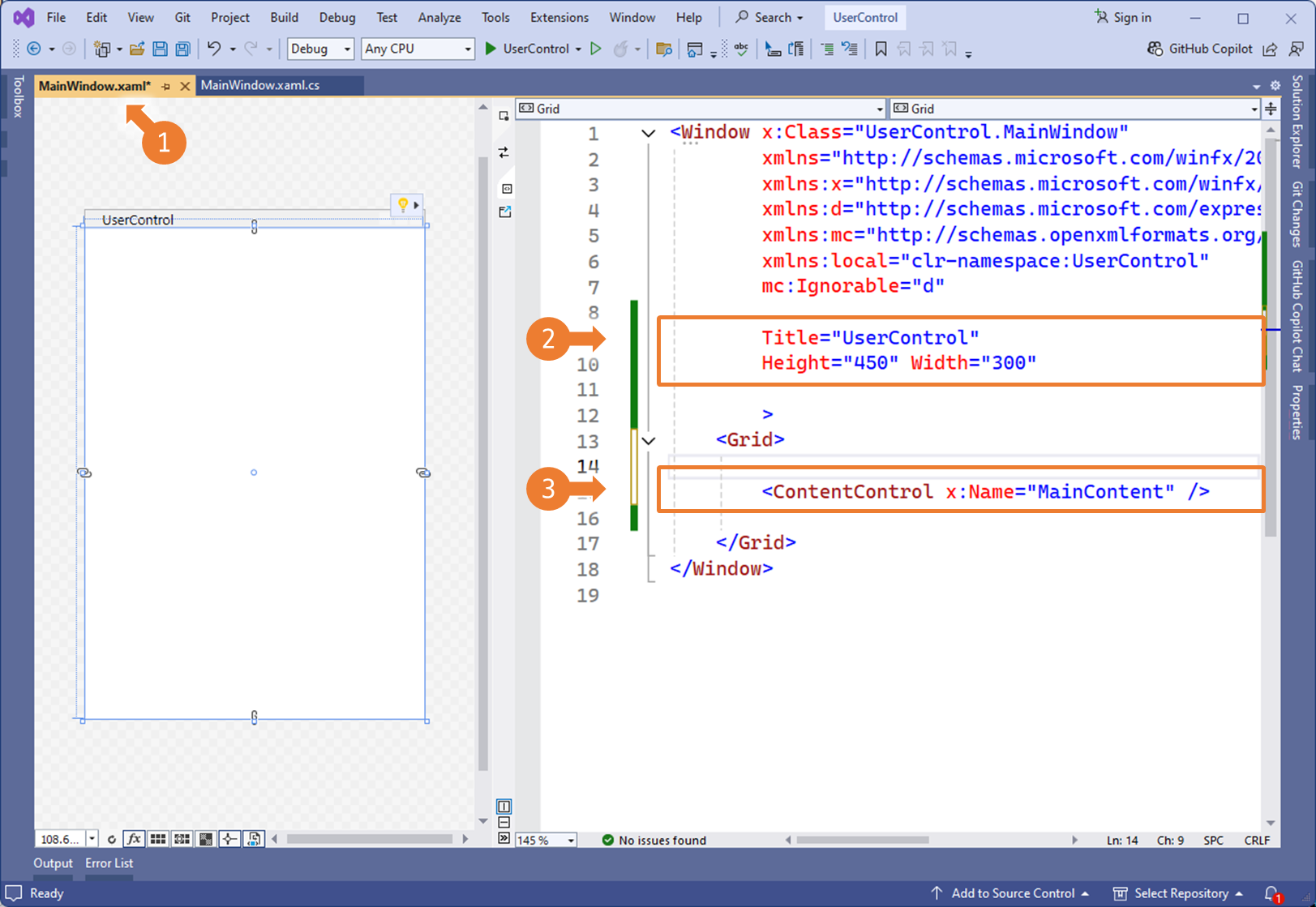
① 프로젝트를 생성하면, 최초 MainWindow 창이 생성됩니다.
② 높이와 너비를 설정하고,
Title="UserControl"
Height="450" Width="300"
③ <Grid> 태그 내에 <ContentControl> 태그를 추가합니다.
<ContentControl x:Name="MainContent" />
02.2 LoginView.xaml 이라는 이름으로 UserControl 파일을 추가
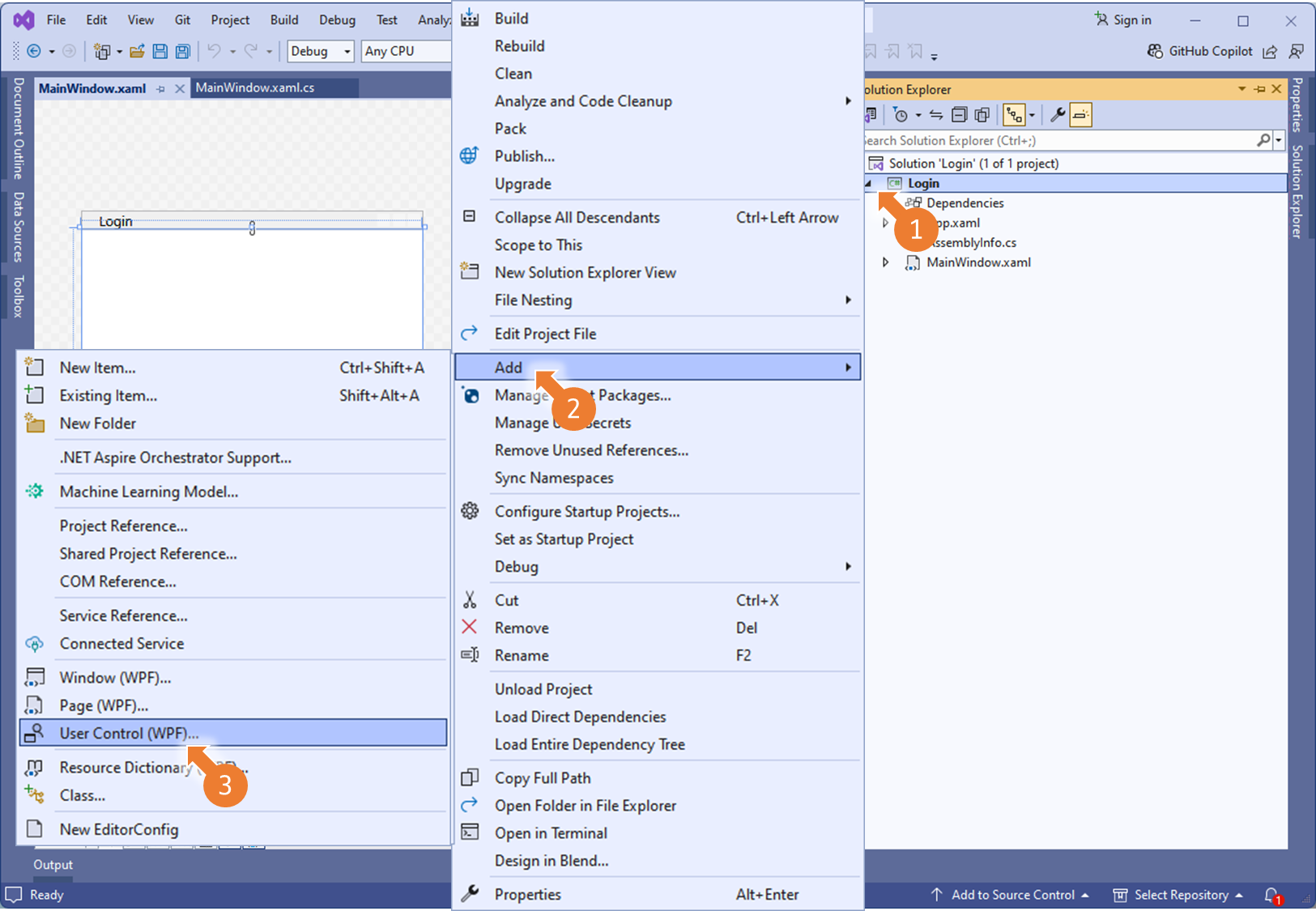
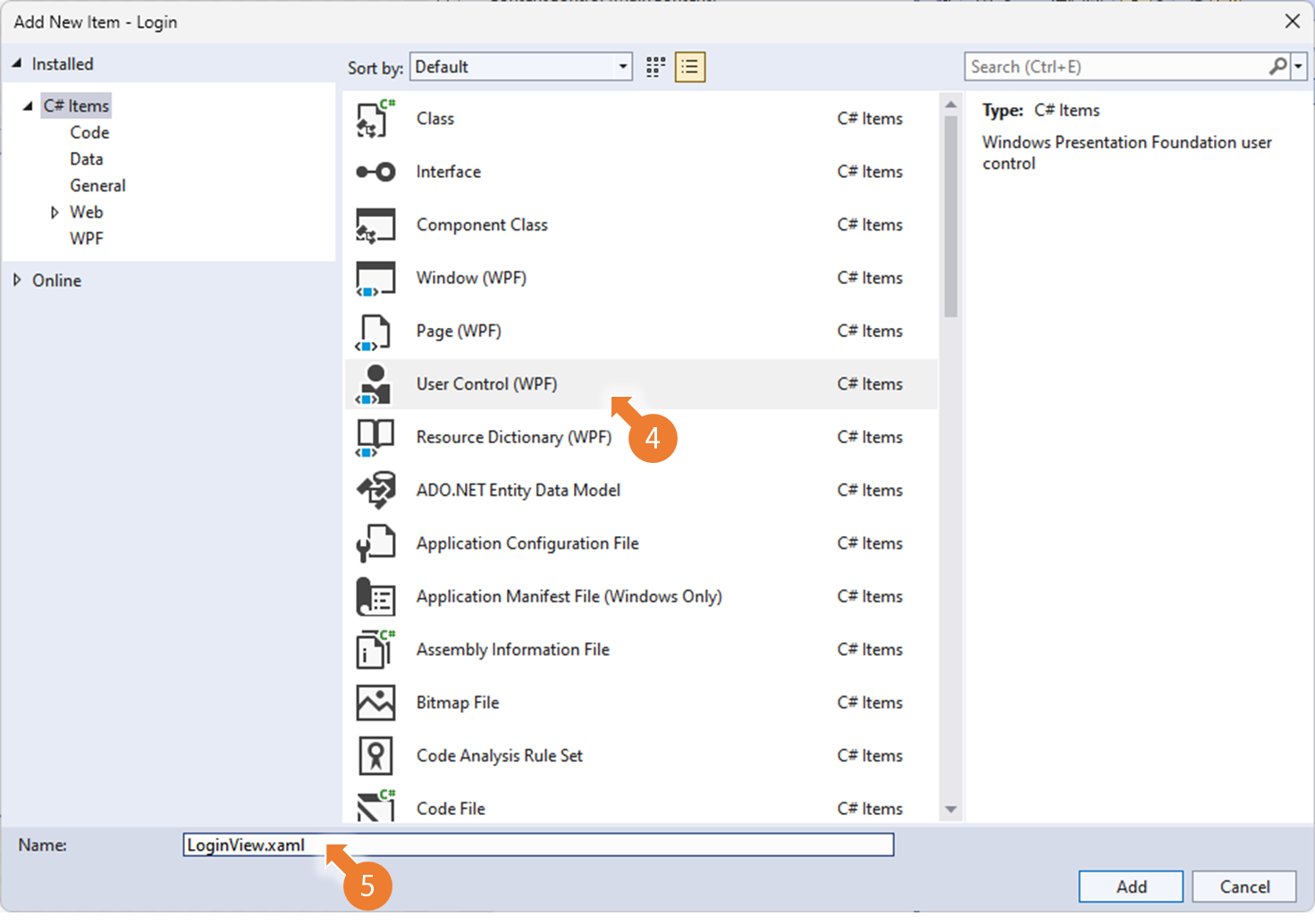
02.3 레이아웃 세팅
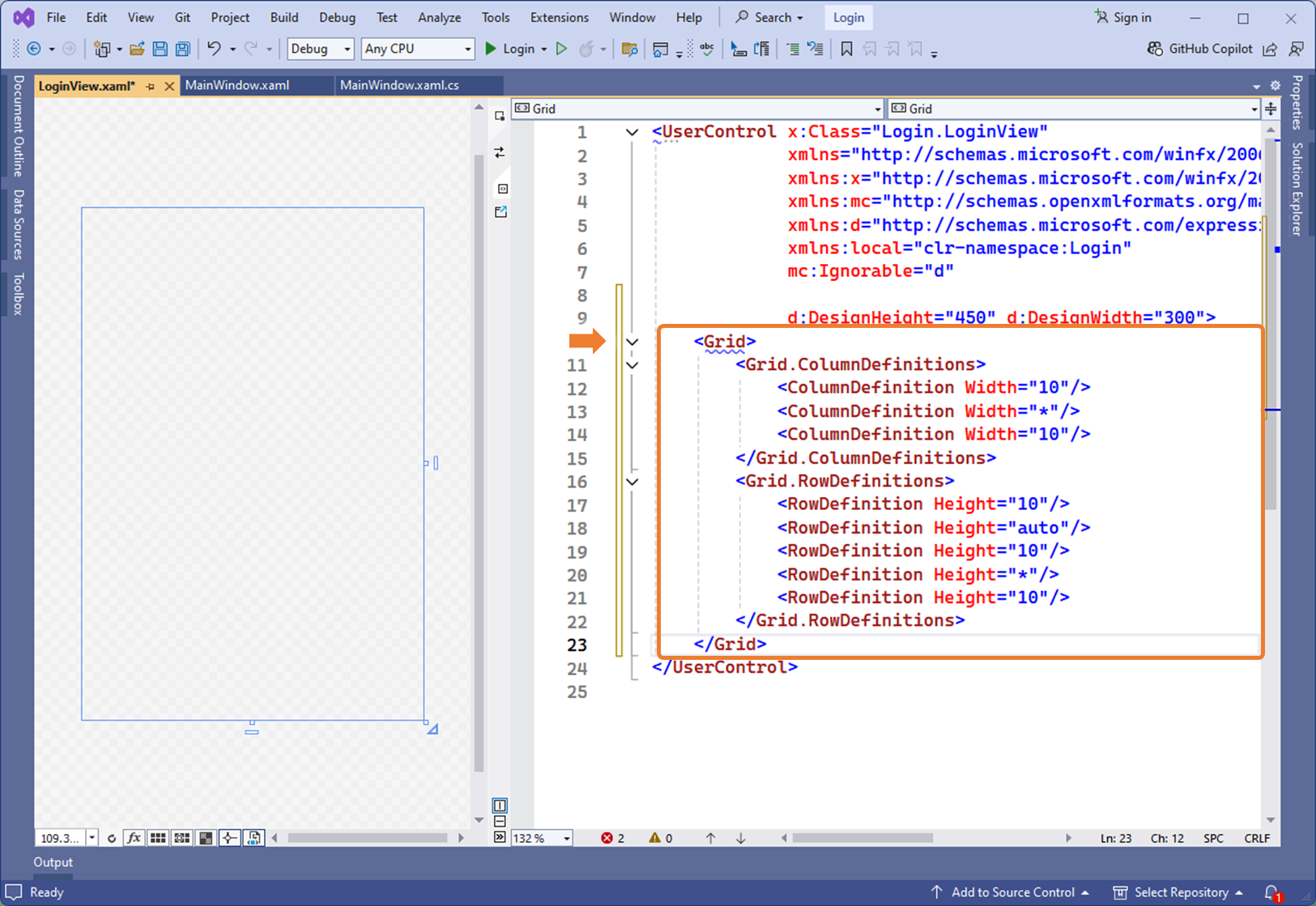
대략적인 그리드를 설정합니다.
d:DesignHeight="450"
d:DesignWidth="300"
<Grid.ColumnDefinitions>
<ColumnDefinition Width="10"/>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="10"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="10"/>
<RowDefinition Height="*"/>
<RowDefinition Height="10"/>
<RowDefinition Height="*"/>
<RowDefinition Height="10"/>
<RowDefinition Height="*"/>
<RowDefinition Height="10"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
02.4 컨트롤 세팅
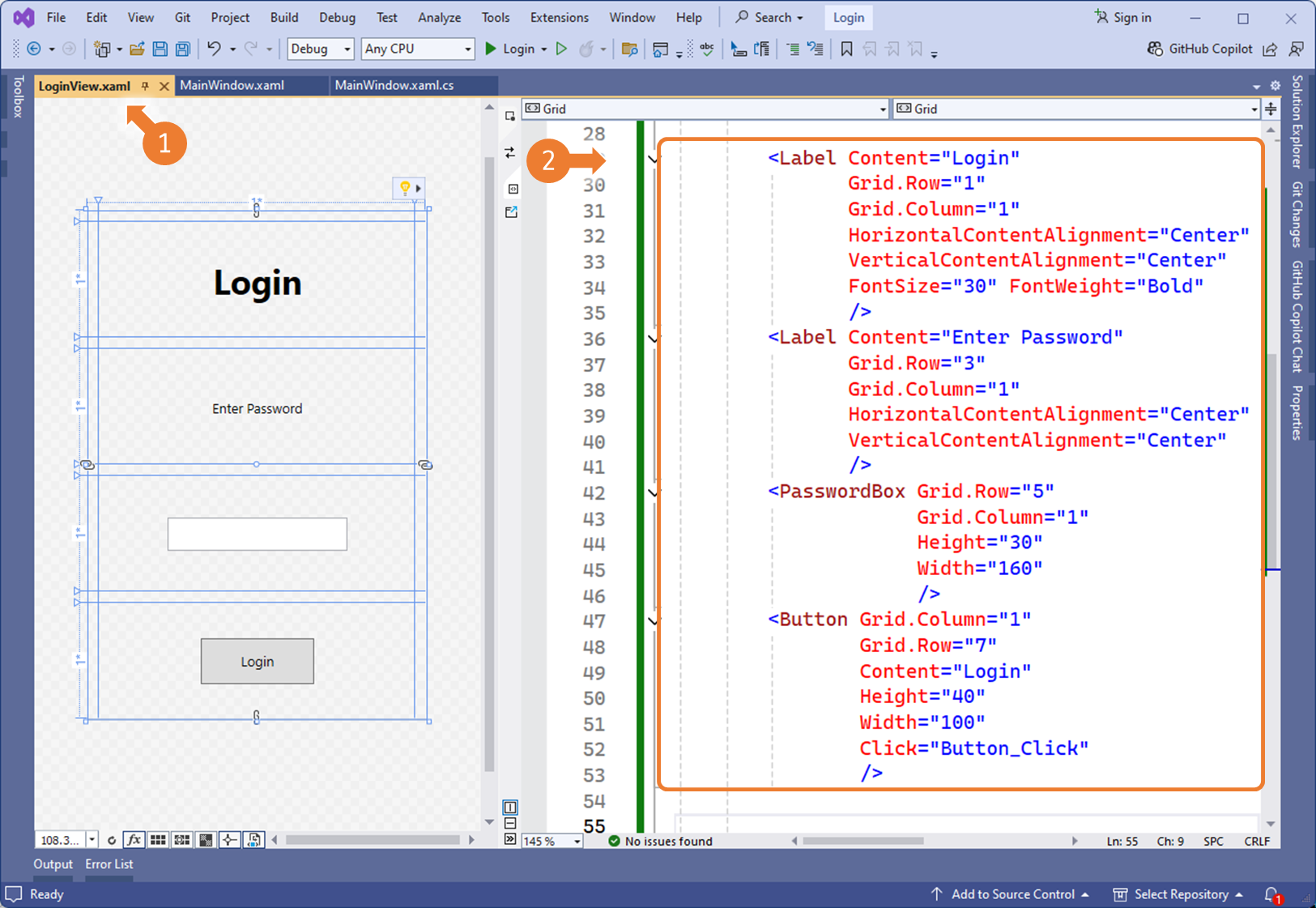
레이블과 비밀번호 입력 박스, 버튼을 추가합니다.
<Label Content="Login"
Grid.Row="1"
Grid.Column="1"
HorizontalContentAlignment="Center"
VerticalContentAlignment="Center"
FontSize="30" FontWeight="Bold"
/>
<Label Content="Enter Password"
Grid.Row="3"
Grid.Column="1"
HorizontalContentAlignment="Center"
VerticalContentAlignment="Center"
/>
<PasswordBox Grid.Row="5"
Grid.Column="1"
Height="26"
Width="160"
/>
<Button Grid.Column="1"
Grid.Row="7"
Content="Login"
Height="40"
Width="100"
/>
02.5
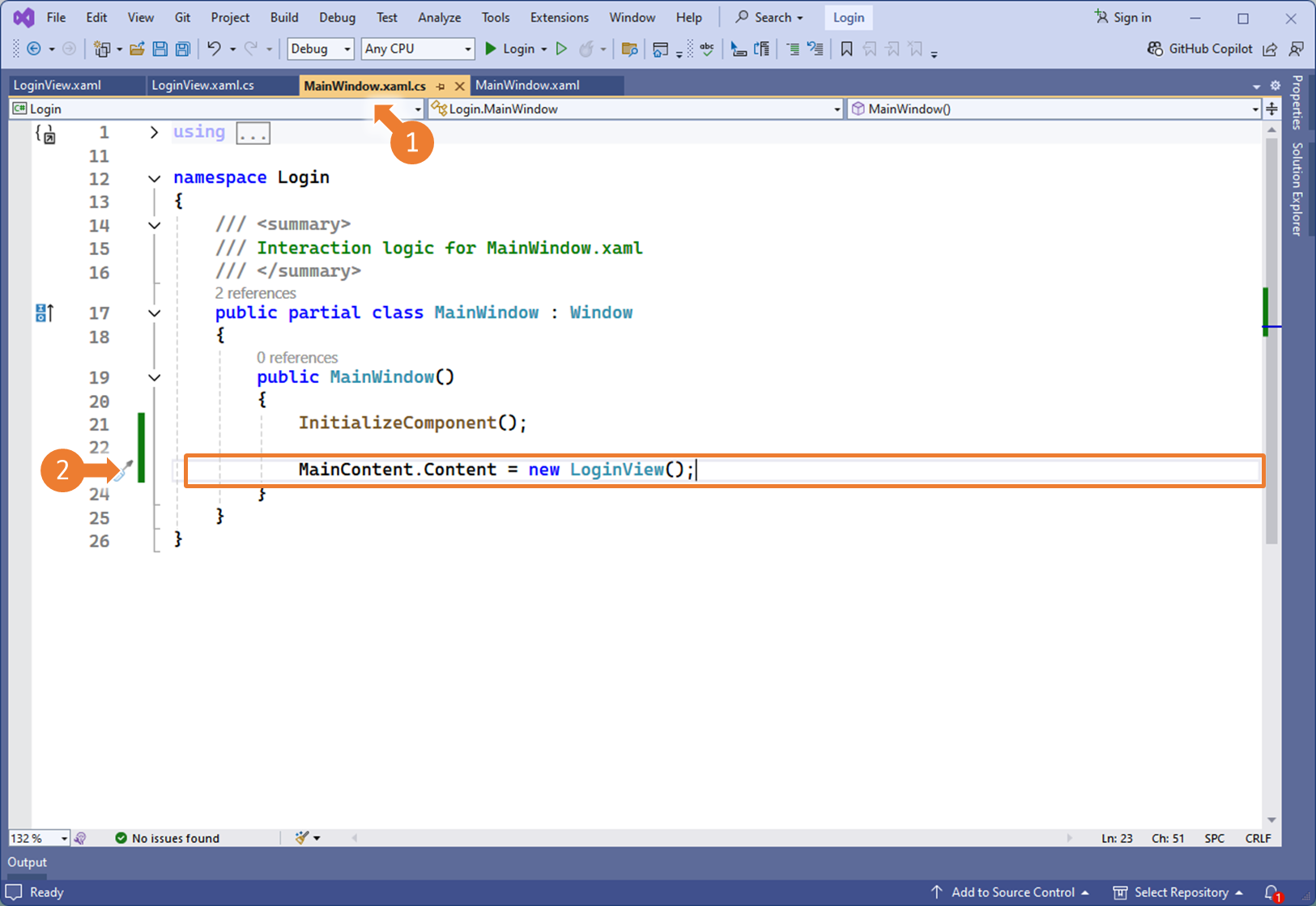
① MainWindow 코드에서, ② MainContent.Content 에 LoginView 컨트롤 객체를 할당합니다.
MainContent.Content = new LoginView();
02.6
빌드 후, 실행하면, MainWindow에서 LoginView 를 가져와 content 로 출력함을 알 수 있습니다.
만약, LoginView.xaml의 d:DesignHeight="450" d:DesignWidth="300" 속성값을
MainWindow 와 다르게, 줄이거나 늘리면 어떻게 되는지도 확인합니다.
03. TodoApp을 UserControl에 복사하기
03.1 이전 포스트에서 만들어 본 TodoApp을 이번 프로젝트에서 UserControl에 복사합니다.
d:DesignHeight="450" d:DesignWidth="300"
03.2 TodoApp.xaml
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="10"/>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="10"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="10"/>
<RowDefinition Height="*"/>
<RowDefinition Height="10"/>
<RowDefinition Height="75"/>
<RowDefinition Height="10"/>
<RowDefinition Height="50"/>
<RowDefinition Height="10"/>
</Grid.RowDefinitions>
<ScrollViewer Grid.Row="1"
Grid.Column="1"
VerticalScrollBarVisibility="Auto"
>
<StackPanel x:Name="TodoList"
Background="{DynamicResource {x:Static SystemColors.HighlightBrushKey}}"/>
</ScrollViewer>
<TextBox x:Name="TodoInput"
Grid.Row="3"
Grid.Column="1"
Background="{DynamicResource {x:Static SystemColors.ActiveCaptionBrushKey}}"
Foreground="white"
/>
<Button Grid.Row="5"
Grid.Column="1"
Content="Add Todo" FontWeight="Bold" FontSize="14" Click="Button_Click"
/>
</Grid>
03.3 TodoApp.xaml.cs
private void Button_Click(object sender, RoutedEventArgs e)
{
string todoText = TodoInput.Text;
if (!string.IsNullOrEmpty(todoText))
{
TextBlock todoItem = new TextBlock
{
Text = todoText
};
TodoList.Children.Add(todoItem);
TodoInput.Clear();
}
}
04. 화면 전환 구현
04.1 로그인 버튼을 눌렀을 때, LoginView에서 TodoApp 으로 전환 로직 구현
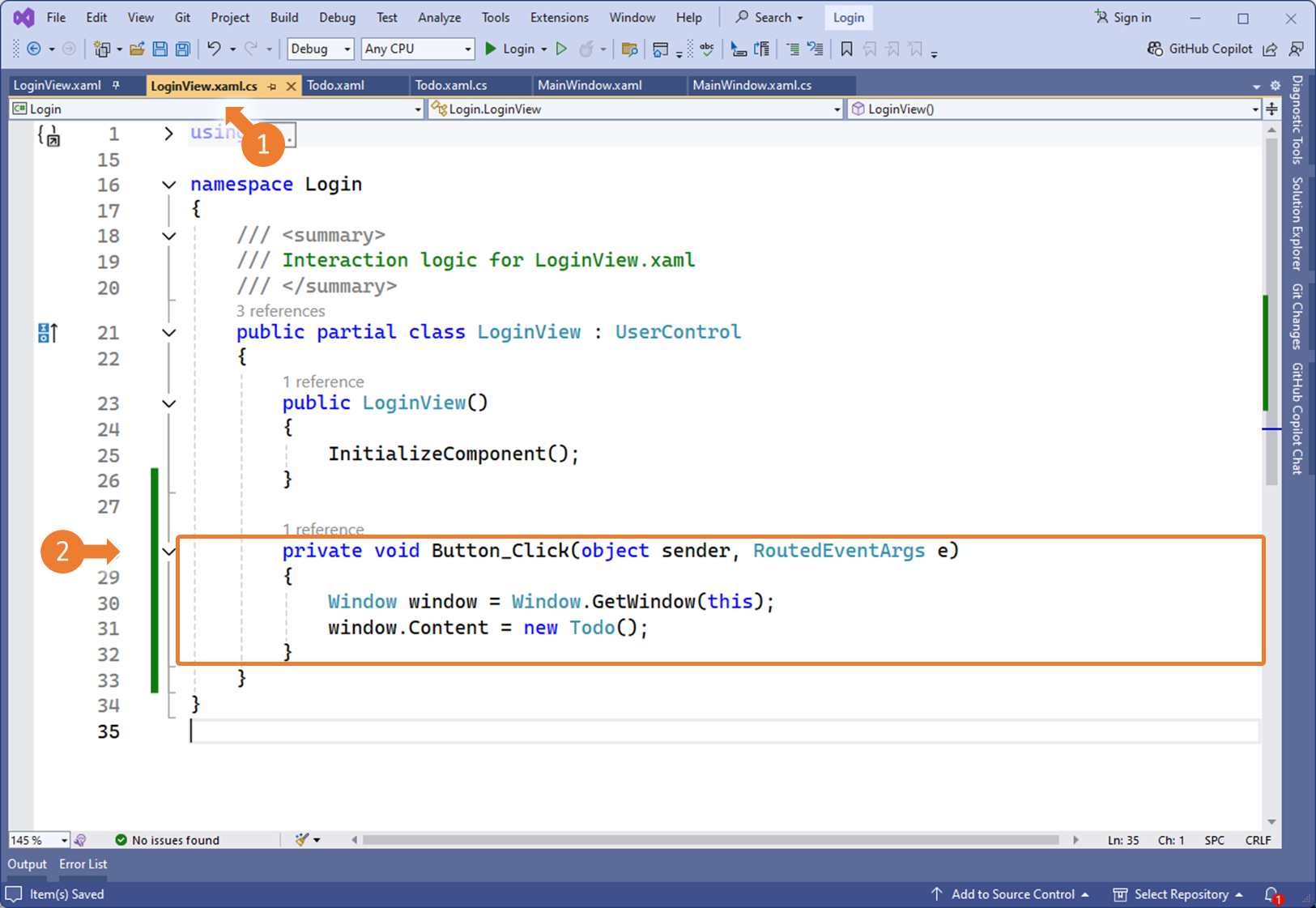
private void Button_Click(object sender, RoutedEventArgs e)
{
Window window = Window.GetWindow(this);
window.Content = new Todo();
}
05. 실행 결과 확인